Here’s an example of using an Interrupt Service Routine (ISR) in MicroPython on a GPIO pin for the Raspberry Pi Pico. In this example, the ISR will trigger when a push button is pressed, toggling an LED each time the button is pushed.
Circuit Diagram
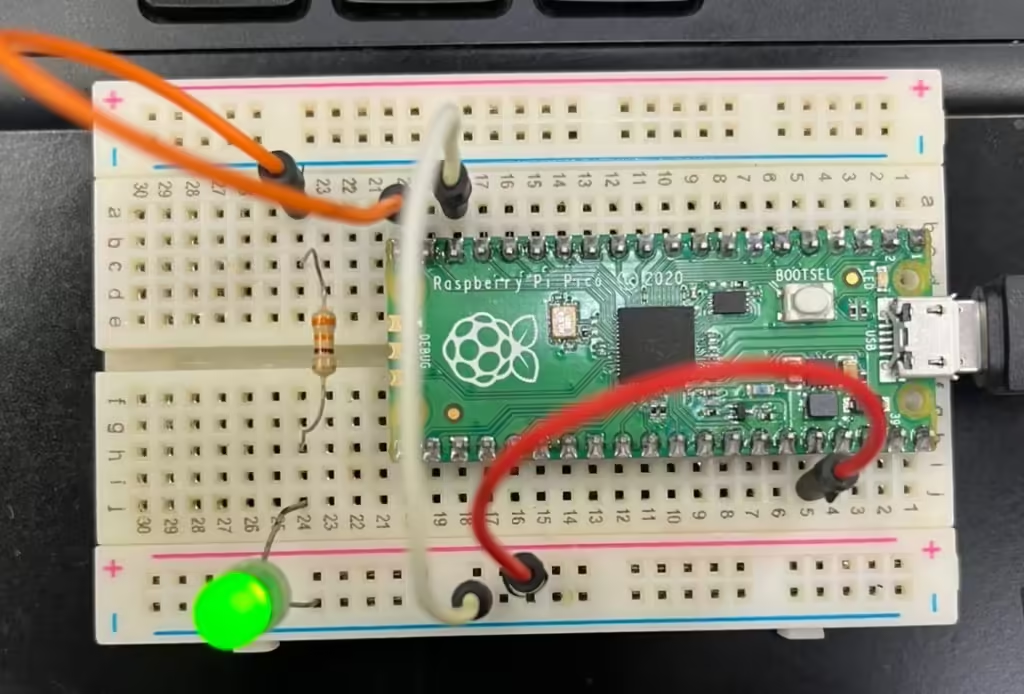
Code: Button Interrupt to Toggle LED
from machine import Pin # Import the Pin class
import time
# Initialize LED on GPIO 15
led = Pin(15, Pin.OUT)
# Initialize Button on GPIO 14 with pull-down resistor
button = Pin(14, Pin.IN, Pin.PULL_DOWN)
# Define an ISR function for the button press
def toggle_led(pin):
led.value(not led.value()) # Toggle the LED state
# Attach the ISR to the button with a rising edge trigger (when button is pressed)
button.irq(trigger=Pin.IRQ_RISING, handler=toggle_led)
# Main loop
while True:
time.sleep(1) # Keep the main loop running
To know how to configure GPIOs for INPUT and OUTPUT, Click Here
To understand how ISR or Interrupt Handler or IRQ Works, Click Here
Explanation
- Pin Setup:
led = Pin(15, Pin.OUT)
: Configures GPIO 15 as an output pin for the LED.button = Pin(14, Pin.IN, Pin.PULL_DOWN)
: Sets up GPIO 14 as an input pin with a pull-down resistor to ensure a stable low state when the button is not pressed.
- ISR Function:
def toggle_led(pin)
: Defines the ISR functiontoggle_led
that toggles the LED’s state by changing its value (led.value(not led.value())
).
- Attach ISR to Button:
button.irq(trigger=Pin.IRQ_RISING, handler=toggle_led)
: Attaches the ISR to trigger on a rising edge (button press). When triggered, it callstoggle_led
.
This code will toggle the LED on and off each time the button is pressed. The irq
function allows for efficient, real-time response to input changes without constant polling.
About Interrupt Service Routine (ISR)
An Interrupt Service Routine (ISR) is a function that responds immediately to specific events, such as a button press, without needing to check for these events continuously in the main program loop. ISRs are commonly used in embedded programming to handle real-time events efficiently.
How ISR Works
- Triggering: ISRs are triggered by hardware or software events, such as a change in a GPIO pin’s state. For instance, in the Raspberry Pi Pico, you can set an ISR to trigger when a button is pressed (rising edge) or released (falling edge).
- Response: Once the ISR is triggered, the microcontroller temporarily pauses the main program and executes the ISR function. This allows the program to respond to the event immediately.
- Execution: ISRs are designed to be quick. They handle tasks like toggling an LED, changing a variable’s state, or signaling other parts of the program, allowing the main loop to proceed efficiently without delays.
- Returning Control: After the ISR function completes, the microcontroller returns to where it left off in the main program, resuming normal operations.
In MicroPython, ISRs on the Raspberry Pi Pico are often set up using the irq()
method, which allows GPIO pins to detect and react to specific trigger events, enhancing the responsiveness of the system without busy-waiting.
Why needs Interrupt Service Routine (ISR) def toggle_led(pin) the parameter (pin)?
Whats type of pin?
Value of (pin)?
Hi Rudolf,
In this particular code, the pin parameter is not explicitly used inside toggle_led. However, it is included by convention to maintain flexibility. For example, you could use pin.value() to check the state of the “button” pin if needed with printf function inside ISR. So pin is infact “button” pin that trigered that particular ISR.