Introduction
The Raspberry Pi Pico W is a microcontroller board that brings Wi-Fi connectivity to the already popular Pico family. By combining the power of this versatile board with the AccuWeather API, you can create projects that fetch real-time weather data and utilize it in innovative ways. This article provides an in-depth guide on how the Raspberry Pi Pico W can connect to the AccuWeather API, retrieve weather data, and showcase its use in various applications.
How APIs Work: An Overview
APIs (Application Programming Interfaces) serve as bridges between software applications, allowing them to exchange data seamlessly. APIs use predefined endpoints and protocols to enable communication. For instance, a weather API allows your program to query weather servers and receive structured data such as temperature, humidity, and wind speed in return.
AccuWeather API: How It Works
The AccuWeather API is a powerful tool for accessing comprehensive weather data. By using your unique API key, you can request data for a specific location, and the API responds with detailed weather conditions, including temperature, wind speed, humidity, and visibility. Requests are made using HTTP GET methods, and the responses are typically in JSON format, making it easy to parse and use in your application.
To fetch data from the AccuWeather API, you’ll need:
- An API key (provided upon registering for AccuWeather’s developer services).
- A location key (specific to your city or region).
- The API endpoint URL with your query parameters.
AccuWeather API Registration: Free Tier
The AccuWeather API is a powerful tool for accessing comprehensive weather data. By using your unique API key, you can request data for a specific location, and the API responds with detailed weather conditions, including temperature, wind speed, humidity, and visibility. Requests are made using HTTP GET methods, and the responses are typically in JSON format, making it easy to parse and use in your application.
To fetch data from the AccuWeather API, you’ll need:
- Open AccuWeather APIs | home
Click on Register to Sign Up for Accuweather account.
Login once Sign Up is Successful.
Click on “MY APPS” tab, Add a new App.
- App is created now.
- Click on the App to see API Key.
- To get
LOCATION_KEY
, ClickAPI REFERENCE
>>Locations API
>>City Search
- Enter Query Parameters “apikey” & “q” as shown below and click
Send This Request
- Query Response will be following. Note down the “Key” (
LOCATION_KEY)
. - To get API URL or Base URL. Click
API REFERENCE
>>Current Conditions API
>>Current Conditions
- Fill in
location key
apikey
,language
,detail
text box as follow and pressSend This Request
. UndercURL
,BASE_URL
will appear.
The Hardware: Raspberry Pi Pico W
Overview of the Pico W
The Raspberry Pi Pico W is an affordable microcontroller board based on the RP2040 chip. It is similar to the original Pico but adds a Wi-Fi module, making it perfect for IoT projects. The board is compact, efficient, and capable of handling wireless communication tasks seamlessly.
Wi-Fi Connectivity
The Pico W features an Infineon CYW43439 Wi-Fi chip, which supports 2.4GHz Wi-Fi networks. This chip enables the Pico W to connect to a wireless network, making it suitable for projects requiring real-time data fetching, such as weather updates from the AccuWeather API.
The Code
Below is a Python script using MicroPython to fetch weather data from the AccuWeather API:
import network # Module for Wi-Fi connectivity
import urequests # Module for making HTTP requests
import time # Module for time delays
import json # Module for handling JSON data
# Wi-Fi credentials
SSID = "YourWiFiSSID" # Replace with your Wi-Fi network name
PASSWORD = "YourWiFiPassword" # Replace with your Wi-Fi password
# AccuWeather API details
API_KEY = "YourAPIKey" # Replace with your API key
LOCATION_KEY = "YourLocationKey" # Replace with your location key
LANGUAGE = "en-us" # Language for the API response
BASE_URL = f"http://dataservice.accuweather.com/currentconditions/v1/{LOCATION_KEY}?apikey={API_KEY}&language={LANGUAGE}&details=true"
def connect_wifi():
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
wlan.connect(SSID, PASSWORD)
print("Connecting to Wi-Fi...")
while not wlan.isconnected():
time.sleep(1)
print("Connected to Wi-Fi!")
print(f"IP address: {wlan.ifconfig()[0]}")
def parse_weather_data(response_data):
if response_data:
weather = response_data[0]
local_time = weather["LocalObservationDateTime"]
weather_text = weather["WeatherText"]
temperature = weather["Temperature"]["Metric"]["Value"]
humidity = weather["RelativeHumidity"]
print("\nWeather Information:")
print(f"Local Time: {local_time}")
print(f"Weather: {weather_text}")
print(f"Temperature: {temperature}°C")
print(f"Humidity: {humidity}%")
else:
print("No weather data available!")
def get_weather():
try:
print("Fetching weather data...")
response = urequests.get(BASE_URL)
if response.status_code == 200:
data = response.json()
parse_weather_data(data)
else:
print(f"Error: HTTP {response.status_code}")
response.close()
except Exception as e:
print(f"Error fetching weather data: {e}")
def main():
connect_wifi()
while True:
get_weather()
time.sleep(5) # Fetch data every 5 seconds
if __name__ == "__main__":
main()
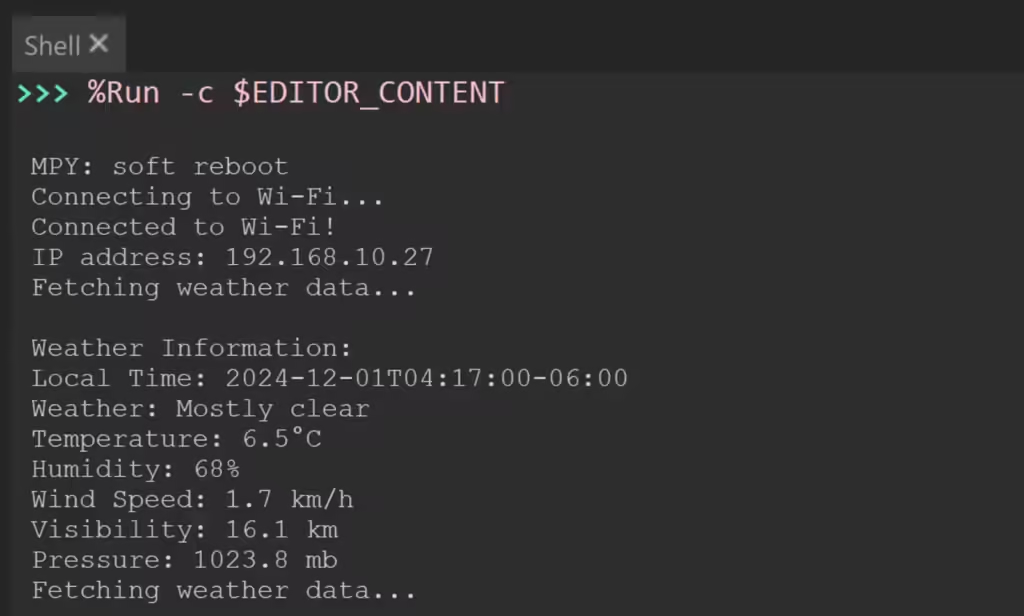
Code Explanation
- Wi-Fi Connection:
- The
connect_wifi()
function activates the Wi-Fi module on the Pico W and connects to the specified network using the given credentials.
- The
- API Request:
- The
get_weather()
function sends an HTTP GET request to the AccuWeather API using theurequests
library. The response is parsed if the request is successful.
- The
- Weather Data Parsing:
- The
parse_weather_data()
function extracts key weather parameters like temperature, humidity, and weather description from the JSON response and prints them.
- The
- Main Loop:
- The
main()
function connects to Wi-Fi and continuously fetches and displays weather data every 5 seconds.
- The
Use Cases for This Project
- Home Automation:
- Integrate weather data into a smart home system for controlling HVAC devices or curtains based on real-time conditions.
- Agriculture:
- Use weather data to assist farmers in making decisions about irrigation and crop management.
- Education:
- Demonstrate IoT and API concepts to students in a hands-on manner.
- Personal Weather Station:
- Combine the project with sensors to create a more comprehensive weather station.
- Travel Advisory Systems:
- Display weather conditions at specific destinations for travelers.
Summary
By leveraging the Wi-Fi capabilities of the Raspberry Pi Pico W and the powerful AccuWeather API, you can create a robust weather-fetching system. This project not only demonstrates the potential of IoT in real-world applications but also serves as an excellent introduction to using APIs and microcontrollers for data-driven projects. Whether for education, automation, or personal use, the possibilities are endless!